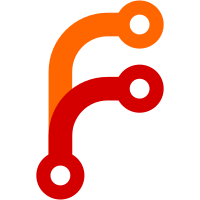
Depending on the browser in different situations the root node itself is selected and new text ends up in a text node on root level instead of a new paragraph. This happens in: * Firefox: after inserting a closed block like a horizontal rule * Chromium: after inserting or selecting such a closed block Now instead of inserting a paragraph directly after inserting an HR, the editor simply checks for normal text input inside the root node and wraps the newly written text with a paragraph (and moves the caret to the end of the paragraph because chromium moves it to the beginning of the line)
18 lines
654 B
TypeScript
18 lines
654 B
TypeScript
const { TEXT_NODE, ELEMENT_NODE } = Node
|
|
|
|
export function isTextNode ({ nodeType }: Node): boolean {
|
|
return nodeType === TEXT_NODE
|
|
}
|
|
export function isElementNode ({ nodeType }: Node): boolean {
|
|
return nodeType === ELEMENT_NODE
|
|
}
|
|
export function isEmptyTextNode (node: Node): boolean {
|
|
return isTextNode(node) && (node as CharacterData).data === ''
|
|
}
|
|
export function isRootNode (node: Node): boolean {
|
|
return (node as HTMLElement).contentEditable === 'true'
|
|
}
|
|
export function isRootChild (node: Node): boolean {
|
|
// TODO: maybe use a data attribute or something for saver identification
|
|
return node.parentElement?.contentEditable === 'true'
|
|
}
|